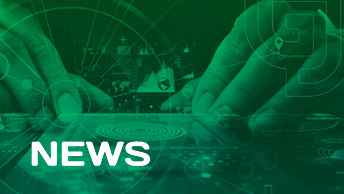
As we discussed yesterday in our post teaching about using native UI overlays with Unity in iOS, we mentioned that our team builds a lot of Unity and native mobile apps. We do so because, when it comes to gaming, augmented reality or virtual reality, it’s one of the best tools possible for showing 3D content.
What we’ve found through our experience is that one of the biggest challenges when using Unity for mobile AR/VR purposes can be providing a user interface that feels inherently native. While Unity 5 improved the UI widget interactions, we find that we’re often left wanting more when compared to native UI widgets.
We won’t settle for a ‘meh’ or even a ‘huh. not bad!’ user experience, so the Gravity Jack team went on a quest, investigating how we can best integrate the native UI widgets with a Unity scene. After all, it’s all about creating the future experience™!
There were just a couple specifications: Our team required a solution that is easy to maintain and that will work with our automated build pipeline. Additionally, it must work on both iOS and Android platforms (obviously).
If you’re looking for iOS help, here’s a tutorial tailored for that. With this post, we’re looking at Android!
Android Native UI Overlay for Android
1) First you will need to create an Android Studio library project. Unfortunately Android Studio doesn’t have an option for this when creating a new project. To make a library project create a new project then edit the build.gradle for the “app” module (not the one in the root project). Change the line “apply plugin: ‘com.android.application’” to “apply plugin: ‘com.android.library’”. Also remove the applicationId property (in the android.default config block) from this file. Here is what it looks like after the changes:
apply plugin: 'com.android.application'
android {
compileSdkVersion 23
buildToolsVersion "23.0.2"
defaultConfig {
minSdkVersion 15
targetSdkVersion 23
versionCode 1
versionName "1.0"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
testCompile 'junit:junit:4.12'
compile 'com.android.support:appcompat-v7:23.1.0'
}
2) Once this is done you need to add the unity classes to this project. On Mac OS X copy /Applications/Unity.app/Contents/PlaybackEngines/AndroidPlayer/Variations/mono/Development/Classes/classes.jar into the app/libs folder in your new project. If your Unity project is setup to use il2cpp replace Variations/mono with Variations/il2cpp.
Now you can create an Activity that extends UnityPlayerActivity
import com.unity3d.player.UnityPlayerActivity;
public class MyActivity extends UnityPlayerActivity {
}
3) Next create a fragment that you will use as your overlay. I created one called UIOverlayFragment. Add any ui that you want to overlay in your fragment and make sure that your fragment view has a clear background if you want to be able to see Unity underneath.
4) In the onCreate method of your activity add the fragment overlay.
public class MyActivity extends UnityPlayerActivity implements UIOverlayFragment.OnFragmentInteractionListener {
@Override
protected void onCreate(Bundle arg0) {
super.onCreate(arg0);
UIOverlayFragment myOverlayFragment = new UIOverlayFragment();
FragmentTransaction ft = getFragmentManager().beginTransaction();
ft.add(android.R.id.content, myOverlayFragment).commit();
}
}
5) Build your android studio project and get the .aar file from the build directory.
6) Add your .aar file to your Unity project in /Plugins/Android/
NOTE: aar files were first supported by Unity 5. You will need to use a jar file for previous versions of unity.
That should have you good to go! Hope this helps provide great user experiences for you too. Have a question or struggling with a step? Let us know by tweeting us and we’ll be sure to help however we can.
Looking for Native UI integration with Unity for iOS? We’ve got that version here.
Happy coding!